mirror of
https://github.com/3b1b/manim.git
synced 2025-04-13 09:47:07 +00:00
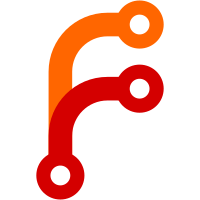
* Comment tweak * Directly print traceback Since the shell.showtraceback is giving some issues * Make InteracrtiveSceneEmbed into a class This way it can keep track of it's internal shell; use of get_ipython has a finicky relationship with reloading. * Move remaining checkpoint_paste logic into scene_embed.py This involved making a few context managers for Scene: temp_record, temp_skip, temp_progress_bar, which seem useful in and of themselves. * Change null key to be the empty string * Ensure temporary svg paths for Text are deleted * Remove unused dict_ops.py functions * Remove break_into_partial_movies from file_writer configuration * Rewrite guarantee_existence using Path * Clean up SceneFileWriter It had a number of vestigial functions no longer used, and some setup that could be made more organized. * Remove --save_pngs CLI arg (which did nothing) * Add --subdivide CLI arg * Remove add_extension_if_not_present * Remove get_sorted_integer_files * Have find_file return Path * Minor clean up * Clean up num_tex_symbols * Fix find_file * Minor cleanup for extract_scene.py * Add preview_frame_while_skipping option to scene config * Use shell.showtraceback function * Move keybindings to config, instead of in-place constants * Replace DEGREES -> DEG * Add arg to clear the cache * Separate out full_tex_to_svg from tex_to_svg And only cache to disk the results of full_tex_to_svg. Otherwise, making edits to the tex_templates would not show up without clearing the cache. * Bug fix in handling BlankScene * Make checkpoint_states an instance variable of CheckpointManager As per https://github.com/3b1b/manim/issues/2272 * Move resizing out of Window.focus, and into Window.init_for_scene * Make default output directory "." instead of "" To address https://github.com/3b1b/manim/issues/2261 * Remove input_file_path arg from SceneFileWriter * Use Dict syntax in place of dict for config more consistently across config.py * Simplify get_output_directory * Swap order of preamble and additional preamble
65 lines
1.6 KiB
Python
65 lines
1.6 KiB
Python
#!/usr/bin/env python
|
|
from addict import Dict
|
|
|
|
from manimlib import __version__
|
|
from manimlib.config import manim_config
|
|
from manimlib.config import parse_cli
|
|
import manimlib.extract_scene
|
|
from manimlib.utils.cache import clear_cache
|
|
from manimlib.window import Window
|
|
|
|
|
|
from IPython.terminal.embed import KillEmbedded
|
|
|
|
|
|
from typing import TYPE_CHECKING
|
|
if TYPE_CHECKING:
|
|
from argparse import Namespace
|
|
|
|
|
|
def run_scenes():
|
|
"""
|
|
Runs the scenes in a loop and detects when a scene reload is requested.
|
|
"""
|
|
# Create a new dict to be able to upate without
|
|
# altering global configuration
|
|
scene_config = Dict(manim_config.scene)
|
|
run_config = manim_config.run
|
|
|
|
if run_config.show_in_window:
|
|
# Create a reusable window
|
|
window = Window(**manim_config.window)
|
|
scene_config.update(window=window)
|
|
|
|
while True:
|
|
try:
|
|
# Blocking call since a scene may init an IPython shell()
|
|
scenes = manimlib.extract_scene.main(scene_config, run_config)
|
|
for scene in scenes:
|
|
scene.run()
|
|
return
|
|
except KillEmbedded:
|
|
# Requested via the `exit_raise` IPython runline magic
|
|
# by means of the reload_scene() command
|
|
pass
|
|
except KeyboardInterrupt:
|
|
break
|
|
|
|
|
|
def main():
|
|
"""
|
|
Main entry point for ManimGL.
|
|
"""
|
|
print(f"ManimGL \033[32mv{__version__}\033[0m")
|
|
|
|
args = parse_cli()
|
|
if args.version and args.file is None:
|
|
return
|
|
if args.clear_cache:
|
|
clear_cache()
|
|
|
|
run_scenes()
|
|
|
|
|
|
if __name__ == "__main__":
|
|
main()
|